JavaScript Security Vulnerabilities and Best Data Protection Practices
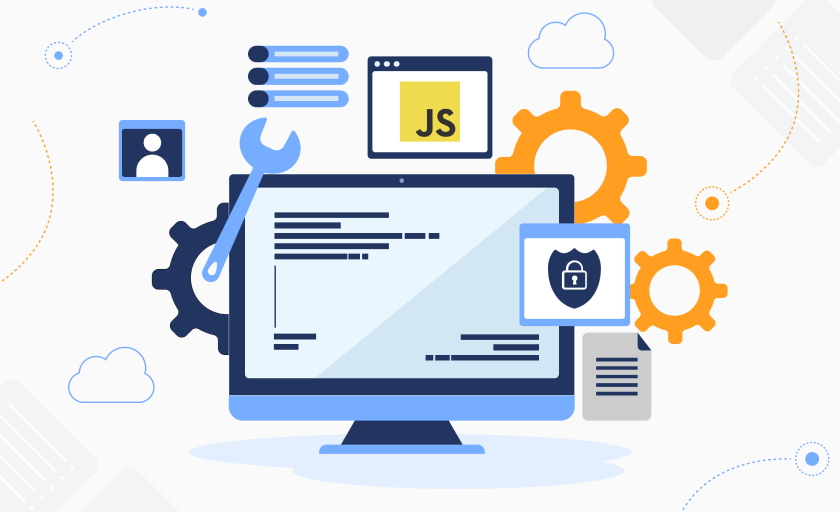
JavaScript is a universal programming language that plays a crucial role in modern web development. However, its flexibility also makes it sensitive to various JavaScript security vulnerabilities. By debugging and implementing strong data protection measures, you can prevent unauthorized access, data leakage, and other security breaches.
The State of Software Security 2023 report mentions a 27% chance that a security vulnerability will be introduced in the first month. At the same time, JavaScript applications, on average, have fewer defects than Java and .NET software. Moreover, those flaws can be resolved faster.
Integrio is a reliable software development company with experienced JavaScript developers. In this article, we will discuss common JavaScript security vulnerabilities and best practices to protect user data and the integrity of your applications.
Common JavaScript Security Vulnerabilities
As a versatile and widely used scripting language, JavaScript presents its own challenges and potential weaknesses. By identifying these vulnerabilities and understanding their implications, you can take proactive steps to fortify your code and protect your users' data from potential threats.
Cross-Site Scripting (XSS)
XSS is a vulnerability that allows an attacker to inject malicious script (usually JavaScript) into web pages viewed by other users. These scripts are executed in the context of the victim's browser, potentially allowing a hacker to steal sensitive data, session cookies, or perform actions on behalf of the victim.
Types of XSS:
Stored XSS. Malicious scripts are permanently stored on the server (in a message on a forum or database) and made available to users.
Reflected XSS. Malicious scripts are embedded in a URL or input field and displayed to users from a web server.
DOM-based XSS. The vulnerability occurs on the client-side resources when scripts directly manipulate the Document Object Model (DOM).
Preventing XSS requires inspecting input data, encoding output data, and implementing Content Security Policy (CSP) headers to limit sources of script execution.
Cross-Site Request Forgery (CSRF)
CSRF is an attack in which a hacker tricks a user into unknowingly making an unwanted request to another website where the victim is authenticated. This way, they can perform actions on the user's behalf without consent.
To prevent CSRF, use anti-CSRF tokens in forms validated on the server. Also, consider applying the SameSite attribute to cookies to limit their use in cross-site requests.
Third-Party Security Vulnerabilities
When your JavaScript code contains third-party libraries or dependencies, you inherit the potential security risks associated with those libraries. These may include outdated versions with known vulnerabilities or malicious code intentionally added by the library's developers.
To mitigate such vulnerabilities, regularly update third-party dependencies and libraries to the latest secure versions. Use tools like npm (Node Package Manager) audit to find and fix weaknesses.
Best Practices for Data Protection in Javascript
It's time to talk about best practices that can significantly improve the security of your JavaScript applications.
Use a JavaScript Linter
JavaScript linter is a tool that analyzes your JavaScript code for potential errors, style issues, and security vulnerabilities.
Detects use of eval(), which can create vulnerabilities
Identifies variables and functions that are defined but never used
Enforces coding standards and formatting rules to facilitate anomaly detection
Detects shadowing of variables, which can lead to undesirable consequences
Ensures that sensitive information is not hard-coded directly into the source code
To follow this practice:
Select a JavaScript linter such as ESLint or JSHint
Configure it with security rules and guidelines
Integrate linter into your development workflow to automatically check code during development and in your continuous integration (CI) pipeline
Audit Dependencies with a Package Manager
Auditing your project's packages is vital to ensure your application has no known security vulnerabilities. You can use package managers like npm or Yarn.
Regularly auditing your dependencies contributes to a secure software supply chain. Ensure you don't accidentally use packages with known vulnerabilities that attackers can exploit.
To apply this practice:
Use the built-in audit or security check commands provided by your package manager (npm audit for npm or yarn audit for Yarn)
Configure automatic checks in your CI/CD pipeline to continuously monitor and report vulnerabilities in your dependencies
Apply updates to your dependencies early to protect your project
Try Subresource Integrity (SRI) Checking
Subresource Integrity is a security feature that allows you to guarantee the integrity and authenticity of external scripts or resources (JavaScript files, stylesheets, or fonts) that your web page downloads from third-party sources like a content delivery network (CDN). This helps prevent attackers from injecting malicious code into these external resources.
How it works:
When you add an external script or resource to your HTML, you include the cryptographic hash of the resource's content as an attribute
When a browser receives a resource, it calculates its hash and compares it to the expected value
If the hashes match, the resource is considered safe and executed
If they don't match, the browser blocks the resource from running, preventing potential security risks
To implement SRI, you add an integrity attribute to your <script> or <link> tags with the appropriate cryptographic hash. Online tools and generators will help you generate the correct hash values.
Establish a Content Security Policy
A Content Security Policy (CSP) allows you to specify which content sources are considered safe and which are not.
When you define a CSP, you declare trusted sources for scripts, styles, images, and other content. You can tell the browser not to execute embedded JavaScript, inline styles, or other potentially dangerous actions. If an attacker tries to inject malicious code into your site, CSP can prevent its execution.
Implementing CSP involves setting HTTP headers on your web server or defining the Content-Security-Policy meta tag in your HTML.
Validate User Input
Always verify that user-submitted data conforms to the expected format, restrictions, and business rules before processing it. It helps you:
Prevent injection attacks such as SQL injection and XSS
Maintain data integrity and prohibit incorrect data from being processed
Improve user interaction so they understand and correct input errors
This practice includes checking data type, length, and format and ensuring compliance with application requirements. For example, if you expect an email address, ensure the data the user enters follows the desired format.
Escape or Encode User Input
Escaping or encoding user input is a primary method of preventing cross-site scripting (XSS) attacks. Convert data into a secure format that can be displayed or stored without exposing your security system.
With this practice, you prevent the execution of malicious scripts in the context of other users' browsers. Proper coding also protects your databases from SQL injection attacks.
To output HTML, use functions such as htmlspecialchars in PHP or escape functions in JavaScript. For database queries, implement parameterized queries or prepared statements to automatically avoid user input.
Don’t Store CSRF Token in Cookies
CSRF attacks occur when a hacker tricks a user into making an unwanted malicious request to a website where the user has authenticated. CSRF tokens will help reduce these risks.
We do not recommend storing the CSRF token in cookies. The fact is that cookies can be vulnerable to theft through XSS attacks. It is better to follow this algorithm:
Create a unique token for each user session and insert it into your HTML forms or headers
When a user submits a form or makes a request, add a token to the request data
On the server, check the token to make sure it matches the expected value.
Ensure Secure Cookie Transmission
Cookies are commonly used to store session and authentication information in web applications. To protect this sensitive data, you should ensure that cookies are securely transmitted over HTTPS and configured with secure attributes.
When cookies are transmitted over an unencrypted HTTP connection, they can be intercepted by attackers using packet sniffers. Use HTTPS to encrypt data transmission between the client and the server.
Secure transfer of cookies prevents eavesdropping and tampering of session data and authentication tokens. In addition, using HTTPS to transfer cookies is recommended by security standards like OWASP.
To implement this practice:
Make sure your web application is served over HTTPS and force HTTP traffic to HTTPS
Use the Secure attribute when setting cookies
Implement HTTP Strict Transport Security (HSTS) to ensure your site is always accessible over HTTPS, even if users first type "http://" in their browsers
Minify, Bundle, and Obfuscate your Code
Minification, bundling, and obfuscation are techniques to optimize and secure your JavaScript code.
Minification is the process of removing spaces, comments, and unnecessary characters from your JavaScript files. This reduces the size of your code, resulting in faster load times for your web pages. Examples of minification tools are UglifyJS, Terser, or Google Closure Compiler.
Bundling involves combining multiple JavaScript files into a single file. This reduces the number of HTTP requests your application makes, improving page load times. Examples of bundling tools are Webpack, Rollup, or Parcel.
Obfuscation makes your code more difficult to understand by renaming variables and functions to non-descriptive names. This helps protect your intellectual property and makes it harder for attackers to reconstruct your code. An example of an obfuscation tool is JavaScript Obfuscator.
After implementing these techniques, thoroughly test your application to ensure that minification, bundling, and obfuscation do not cause functionality or performance issues.
Protect Your JavaScript Solution with Integrio
Developers love JavaScript for its flexibility and versatility but should pay attention to cross-site scripting (XSS), cross-site request forgery (CSRF), and third-party security vulnerabilities. Using a JavaScript linter, dependencies audit, Content Security Policy, CSRF token, and other measures will help you enhance the security posture of your JavaScript applications. Also, you can hire dedicated developers from Integrio to guarantee safety.
Integrio is an app and web development vendor with a proven track record of securing JavaScript software. We create advanced solutions powered by artificial intelligence and machine learning for various industries, including aviation, manufacturing, real estate, telecommunications, transportation, digital marketing, health and fitness, etc.
Do you want to create a secure JavaScript application or hire such a specialist already on your in-house team? Contact us to discuss your project.
Contact us
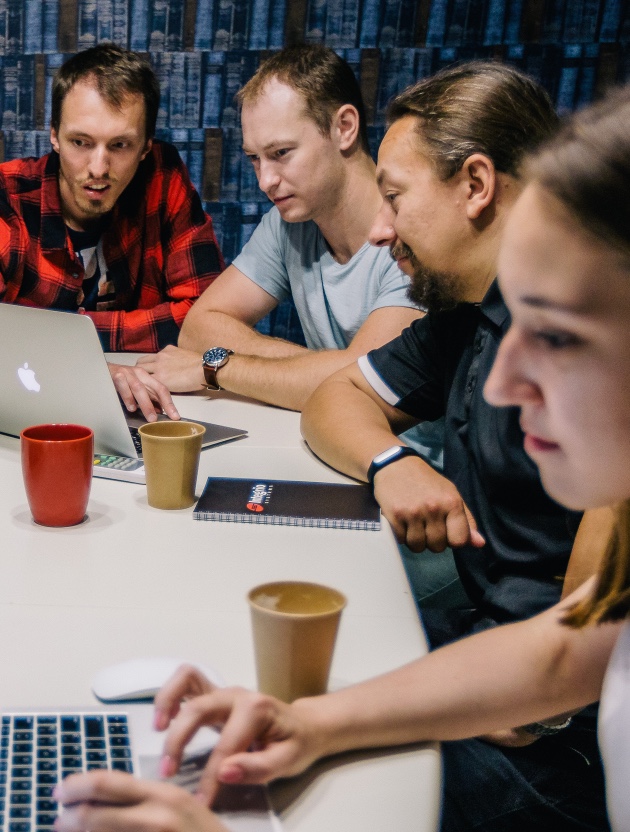